FloatingActionButton
Common
Component in Material Compose
A floating action button (FAB) represents the primary action of a screen.
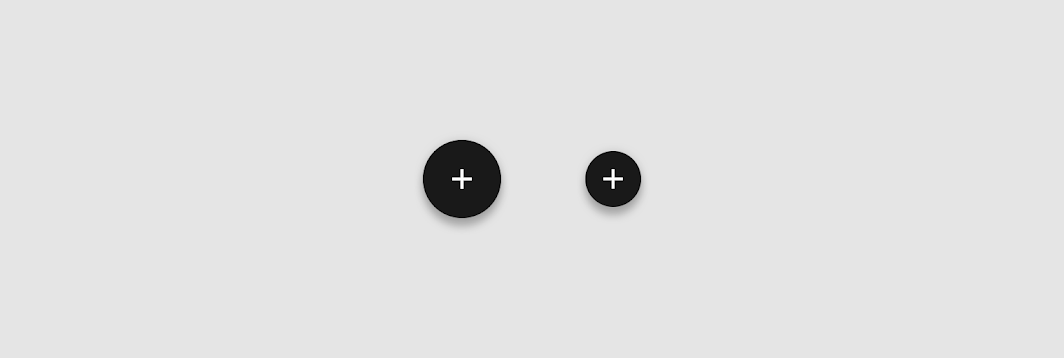
Last updated:
Installation
dependencies {
implementation("androidx.compose.material:material:1.8.0-rc01")
}
Overloads
@OptIn(ExperimentalMaterialApi::class)
@Composable
fun FloatingActionButton(
onClick: () -> Unit,
modifier: Modifier = Modifier,
interactionSource: MutableInteractionSource? = null,
shape: Shape = MaterialTheme.shapes.small.copy(CornerSize(percent = 50)),
backgroundColor: Color = MaterialTheme.colors.secondary,
contentColor: Color = contentColorFor(backgroundColor),
elevation: FloatingActionButtonElevation = FloatingActionButtonDefaults.elevation(),
content: @Composable () -> Unit
)
Parameters
name | description |
---|---|
onClick | callback invoked when this FAB is clicked |
modifier | [Modifier] to be applied to this FAB. |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this FAB. You can use this to change the FAB's appearance or preview the FAB in different states. Note that if null is provided, interactions will still happen internally. |
shape | The [Shape] of this FAB |
backgroundColor | The background color. Use [Color.Transparent] to have no color |
contentColor | The preferred content color for content inside this FAB |
elevation | [FloatingActionButtonElevation] used to resolve the elevation for this FAB in different states. This controls the size of the shadow below the FAB. |
content | the content of this FAB - this is typically an [Icon]. |
Code Example
SimpleFab
@Composable
fun SimpleFab() {
FloatingActionButton(onClick = { /*do something*/ }) {
Icon(Icons.Filled.Favorite, contentDescription = "Localized description")
}
}