LinearProgressIndicator
Common
Component in Material Compose
Progress indicators express an unspecified wait time or display the length of a process.
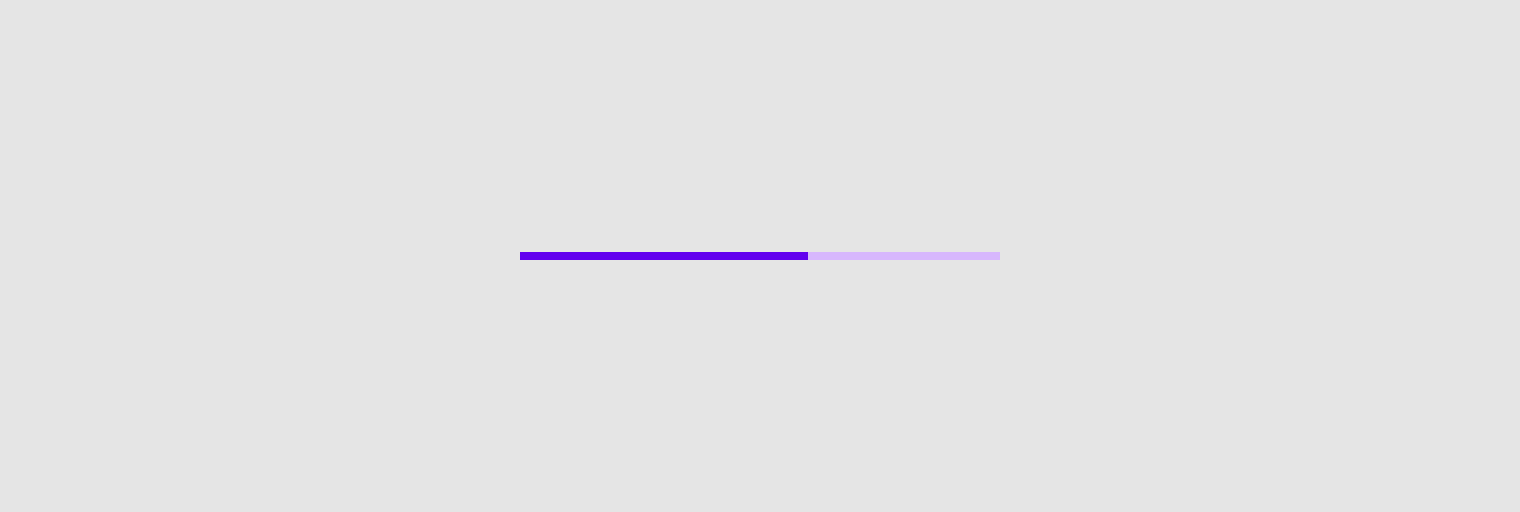
Last updated:
Installation
dependencies {
implementation("androidx.compose.material:material:1.8.0-alpha04")
}
Overloads
@Composable
fun LinearProgressIndicator(
@FloatRange(from = 0.0, to = 1.0) progress: Float,
modifier: Modifier = Modifier,
color: Color = MaterialTheme.colors.primary,
backgroundColor: Color = color.copy(alpha = IndicatorBackgroundOpacity),
strokeCap: StrokeCap = StrokeCap.Butt,
)
Parameters
name | description |
---|---|
progress | The progress of this progress indicator, where 0.0 represents no progress and 1.0 represents full progress. Values outside of this range are coerced into the range. |
modifier | the [Modifier] to be applied to this progress indicator |
color | The color of the progress indicator. |
backgroundColor | The color of the background behind the indicator, visible when the progress has not reached that area of the overall indicator yet. |
strokeCap | stroke cap to use for the ends of this progress indicator |
@Composable
fun LinearProgressIndicator(
modifier: Modifier = Modifier,
color: Color = MaterialTheme.colors.primary,
backgroundColor: Color = color.copy(alpha = IndicatorBackgroundOpacity),
strokeCap: StrokeCap = StrokeCap.Butt,
)
Parameters
name | description |
---|---|
modifier | the [Modifier] to be applied to this progress indicator |
color | The color of the progress indicator. |
backgroundColor | The color of the background behind the indicator, visible when the progress has not reached that area of the overall indicator yet. |
strokeCap | stroke cap to use for the ends of this progress indicator |
@Deprecated("Maintained for binary compatibility", level = DeprecationLevel.HIDDEN)
@Composable
fun LinearProgressIndicator(
progress: Float,
modifier: Modifier = Modifier,
color: Color = MaterialTheme.colors.primary,
backgroundColor: Color = color.copy(alpha = IndicatorBackgroundOpacity)
)
@Deprecated("Maintained for binary compatibility", level = DeprecationLevel.HIDDEN)
@Composable
fun LinearProgressIndicator(
modifier: Modifier = Modifier,
color: Color = MaterialTheme.colors.primary,
backgroundColor: Color = color.copy(alpha = IndicatorBackgroundOpacity)
)
Code Example
LinearProgressIndicatorSample
@Composable
fun LinearProgressIndicatorSample() {
var progress by remember { mutableStateOf(0.1f) }
val animatedProgress by
animateFloatAsState(
targetValue = progress,
animationSpec = ProgressIndicatorDefaults.ProgressAnimationSpec
)
Column(horizontalAlignment = Alignment.CenterHorizontally) {
LinearProgressIndicator(progress = animatedProgress)
Spacer(Modifier.requiredHeight(30.dp))
OutlinedButton(onClick = { if (progress < 1f) progress += 0.1f }) { Text("Increase") }
}
}