TextButton
Common
Component in Material Compose
Text buttons are typically used for less-pronounced actions, including those located in dialogs and cards. In cards, text buttons help maintain an emphasis on card content.
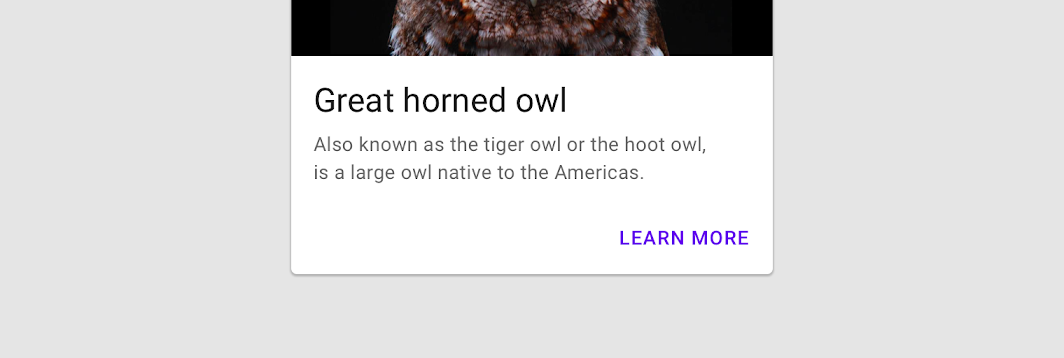
Last updated:
Installation
dependencies {
implementation("androidx.compose.material:material:1.9.0-alpha04")
}
Overloads
@Composable
@NonRestartableComposable
fun TextButton(
onClick: () -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
interactionSource: MutableInteractionSource? = null,
elevation: ButtonElevation? = null,
shape: Shape = MaterialTheme.shapes.small,
border: BorderStroke? = null,
colors: ButtonColors = ButtonDefaults.textButtonColors(),
contentPadding: PaddingValues = ButtonDefaults.TextButtonContentPadding,
content: @Composable RowScope.() -> Unit,
)
Parameters
name | description |
---|---|
onClick | Will be called when the user clicks the button |
modifier | Modifier to be applied to the button |
enabled | Controls the enabled state of the button. When false , this button will not be clickable |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this button. You can use this to change the button's appearance or preview the button in different states. Note that if null is provided, interactions will still happen internally. |
elevation | [ButtonElevation] used to resolve the elevation for this button in different states. A TextButton typically has no elevation, see [Button] for a button with elevation. |
shape | Defines the button's shape as well as its shadow |
border | Border to draw around the button |
colors | [ButtonColors] that will be used to resolve the background and content color for this button in different states. See [ButtonDefaults.textButtonColors]. |
contentPadding | The spacing values to apply internally between the container and the content |
content | The content displayed on the button, expected to be text. |
Code Example
TextButtonSample
@Composable
fun TextButtonSample() {
TextButton(onClick = { /* Do something! */ }) { Text("Text Button") }
}