DatePicker
Common
Component in Material 3 Compose
Date pickers let people select a date and preferably should be embedded into Dialogs. See [DatePickerDialog].
By default, a date picker lets you pick a date via a calendar UI. However, it also allows switching into a date input mode for a manual entry of dates using the numbers on a keyboard.
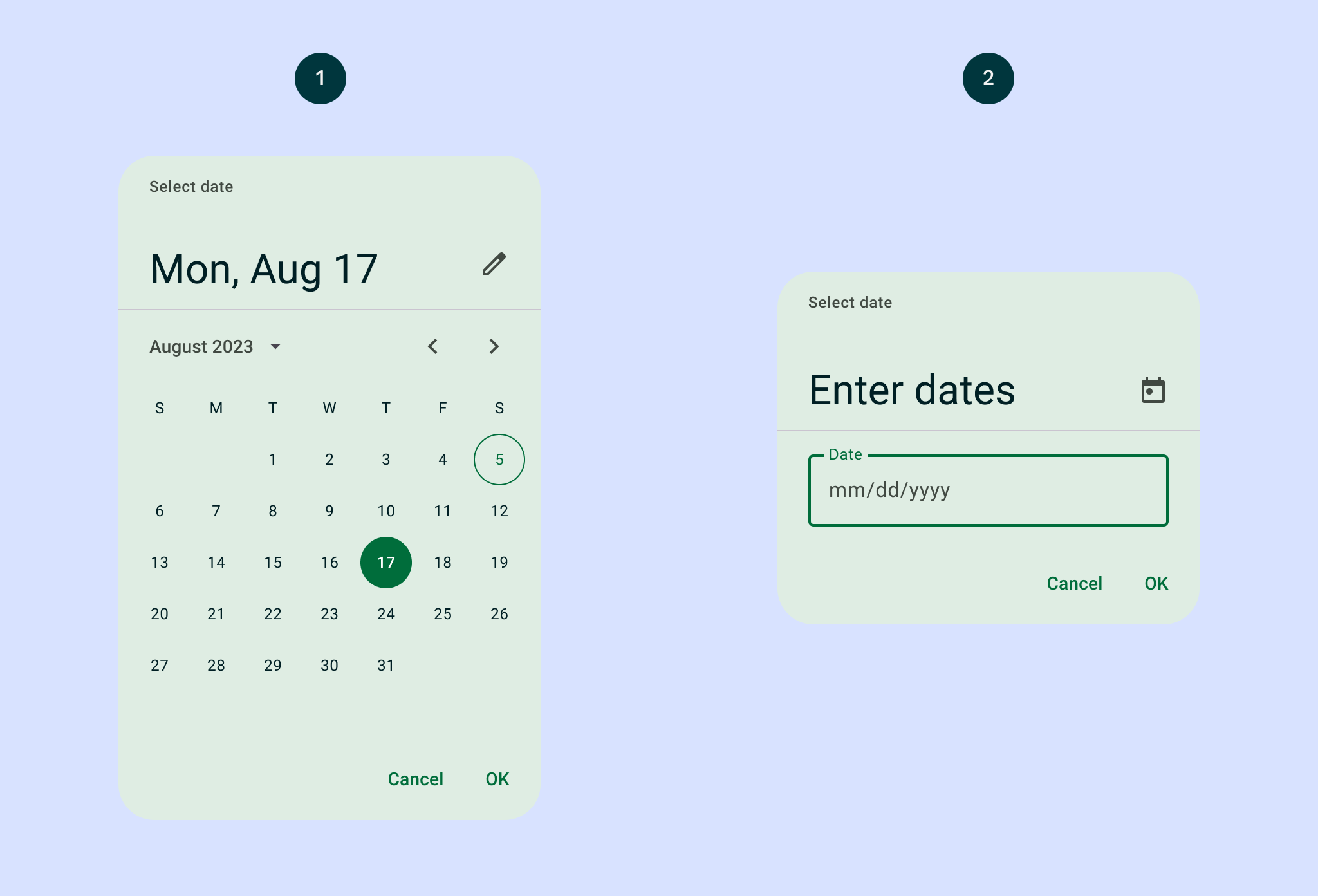
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha02")
}
Overloads
@ExperimentalMaterial3Api
@Composable
fun DatePicker(
state: DatePickerState,
modifier: Modifier = Modifier,
dateFormatter: DatePickerFormatter = remember { DatePickerDefaults.dateFormatter() },
colors: DatePickerColors = DatePickerDefaults.colors(),
title: (@Composable () -> Unit)? = {
DatePickerDefaults.DatePickerTitle(
displayMode = state.displayMode,
modifier = Modifier.padding(DatePickerTitlePadding),
contentColor = colors.titleContentColor
)
},
headline: (@Composable () -> Unit)? = {
DatePickerDefaults.DatePickerHeadline(
selectedDateMillis = state.selectedDateMillis,
displayMode = state.displayMode,
dateFormatter = dateFormatter,
modifier = Modifier.padding(DatePickerHeadlinePadding),
contentColor = colors.headlineContentColor
)
},
showModeToggle: Boolean = true,
)
Parameters
name | description |
---|---|
state | state of the date picker. See [rememberDatePickerState]. |
modifier | the [Modifier] to be applied to this date picker |
dateFormatter | a [DatePickerFormatter] that provides formatting skeletons for dates display |
colors | [DatePickerColors] that will be used to resolve the colors used for this date picker in different states. See [DatePickerDefaults.colors]. |
title | the title to be displayed in the date picker |
headline | the headline to be displayed in the date picker |
showModeToggle | indicates if this DatePicker should show a mode toggle action that transforms it into a date input |
@Deprecated(
message =
"Maintained for binary compatibility. Use the DatePicker with the different" +
" order of parameters.",
level = DeprecationLevel.HIDDEN
)
@ExperimentalMaterial3Api
@Composable
fun DatePicker(
state: DatePickerState,
modifier: Modifier = Modifier,
dateFormatter: DatePickerFormatter = remember { DatePickerDefaults.dateFormatter() },
title: (@Composable () -> Unit)? = {
DatePickerDefaults.DatePickerTitle(
displayMode = state.displayMode,
modifier = Modifier.padding(DatePickerTitlePadding)
)
},
headline: (@Composable () -> Unit)? = {
DatePickerDefaults.DatePickerHeadline(
selectedDateMillis = state.selectedDateMillis,
displayMode = state.displayMode,
dateFormatter = dateFormatter,
modifier = Modifier.padding(DatePickerHeadlinePadding)
)
},
showModeToggle: Boolean = true,
colors: DatePickerColors = DatePickerDefaults.colors()
)
Parameters
name | description |
---|---|
state | state of the date picker. See [rememberDatePickerState]. |
modifier | the [Modifier] to be applied to this date picker |
dateFormatter | a [DatePickerFormatter] that provides formatting skeletons for dates display |
title | the title to be displayed in the date picker |
headline | the headline to be displayed in the date picker |
showModeToggle | indicates if this DatePicker should show a mode toggle action that transforms it into a date input |
colors | [DatePickerColors] that will be used to resolve the colors used for this date picker in different states. See [DatePickerDefaults.colors]. |
Code Examples
DatePickerSample
@OptIn(ExperimentalMaterial3Api::class)
@Preview
@Composable
fun DatePickerSample() {
Column(
modifier = Modifier.verticalScroll(rememberScrollState()),
verticalArrangement = Arrangement.spacedBy(8.dp)
) {
// Pre-select a date for January 4, 2020
val datePickerState = rememberDatePickerState(initialSelectedDateMillis = 1578096000000)
DatePicker(state = datePickerState, modifier = Modifier.padding(16.dp))
Text(
"Selected date timestamp: ${datePickerState.selectedDateMillis ?: "no selection"}",
modifier = Modifier.align(Alignment.CenterHorizontally)
)
}
}
DateInputSample
@OptIn(ExperimentalMaterial3Api::class)
@Preview
@Composable
fun DateInputSample() {
Column(verticalArrangement = Arrangement.spacedBy(8.dp)) {
val state = rememberDatePickerState(initialDisplayMode = DisplayMode.Input)
DatePicker(state = state, modifier = Modifier.padding(16.dp))
Text(
"Entered date timestamp: ${state.selectedDateMillis ?: "no input"}",
modifier = Modifier.align(Alignment.CenterHorizontally)
)
}
}
DatePickerWithDateSelectableDatesSample
@Suppress("ClassVerificationFailure")
@OptIn(ExperimentalMaterial3Api::class)
@Preview
@Composable
fun DatePickerWithDateSelectableDatesSample() {
val datePickerState =
rememberDatePickerState(
selectableDates =
object : SelectableDates {
// Blocks Sunday and Saturday from being selected.
override fun isSelectableDate(utcTimeMillis: Long): Boolean {
return if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val dayOfWeek =
Instant.ofEpochMilli(utcTimeMillis)
.atZone(ZoneId.of("UTC"))
.toLocalDate()
.dayOfWeek
dayOfWeek != DayOfWeek.SUNDAY && dayOfWeek != DayOfWeek.SATURDAY
} else {
val calendar = Calendar.getInstance(TimeZone.getTimeZone("UTC"))
calendar.timeInMillis = utcTimeMillis
calendar[Calendar.DAY_OF_WEEK] != Calendar.SUNDAY &&
calendar[Calendar.DAY_OF_WEEK] != Calendar.SATURDAY
}
}
// Allow selecting dates from year 2023 forward.
override fun isSelectableYear(year: Int): Boolean {
return year > 2022
}
}
)
Column(
modifier = Modifier.verticalScroll(rememberScrollState()),
verticalArrangement = Arrangement.spacedBy(8.dp)
) {
DatePicker(state = datePickerState)
Text(
"Selected date timestamp: ${datePickerState.selectedDateMillis ?: "no selection"}",
modifier = Modifier.align(Alignment.CenterHorizontally)
)
}
}