ElevatedCard
Common
Component in Material 3 Compose
Elevated cards contain content and actions that relate information about a subject. They have a drop shadow, providing more separation from the background than filled cards, but less than outlined cards.
This ElevatedCard does not handle input events - see the other ElevatedCard overloads if you want a clickable or selectable ElevatedCard.
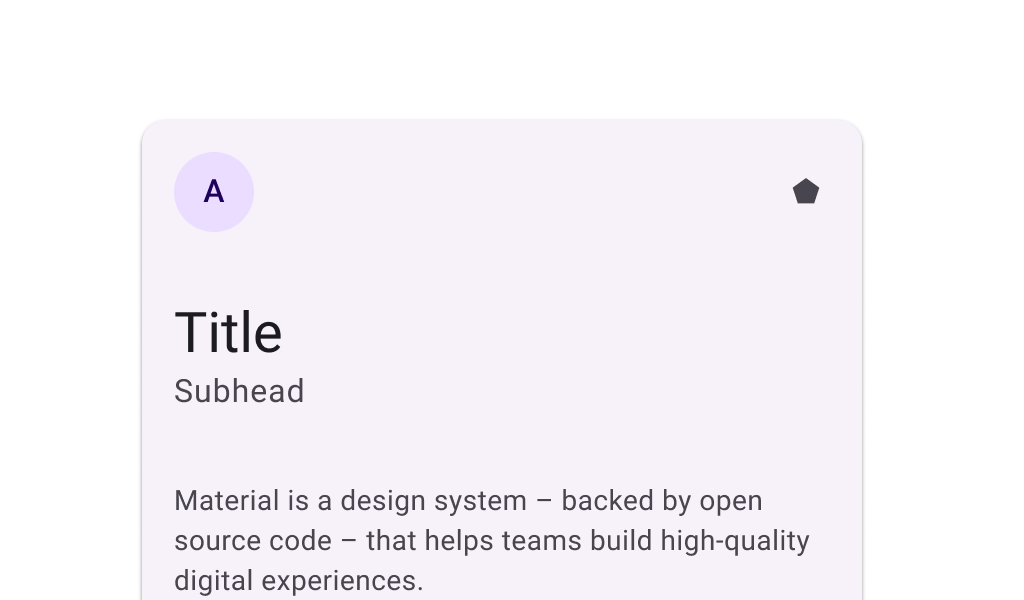
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha12")
}
Overloads
@Composable
fun ElevatedCard(
modifier: Modifier = Modifier,
shape: Shape = CardDefaults.elevatedShape,
colors: CardColors = CardDefaults.elevatedCardColors(),
elevation: CardElevation = CardDefaults.elevatedCardElevation(),
content: @Composable ColumnScope.() -> Unit
)
Parameters
name | description |
---|---|
modifier | the [Modifier] to be applied to this card |
shape | defines the shape of this card's container and shadow (when using [elevation]) |
colors | [CardColors] that will be used to resolve the color(s) used for this card in different states. See [CardDefaults.elevatedCardElevation]. |
elevation | [CardElevation] used to resolve the elevation for this card in different states. This controls the size of the shadow below the card. Additionally, when the container color is [ColorScheme.surface], this controls the amount of primary color applied as an overlay. See also: [Surface]. |
content | The content displayed on the card |
@Composable
fun ElevatedCard(
onClick: () -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
shape: Shape = CardDefaults.elevatedShape,
colors: CardColors = CardDefaults.elevatedCardColors(),
elevation: CardElevation = CardDefaults.elevatedCardElevation(),
interactionSource: MutableInteractionSource? = null,
content: @Composable ColumnScope.() -> Unit
)
Parameters
name | description |
---|---|
onClick | called when this card is clicked |
modifier | the [Modifier] to be applied to this card |
enabled | controls the enabled state of this card. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
shape | defines the shape of this card's container and shadow (when using [elevation]) |
colors | [CardColors] that will be used to resolve the color(s) used for this card in different states. See [CardDefaults.elevatedCardElevation]. |
elevation | [CardElevation] used to resolve the elevation for this card in different states. This controls the size of the shadow below the card. Additionally, when the container color is [ColorScheme.surface], this controls the amount of primary color applied as an overlay. See also: [Surface]. |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this card. You can use this to change the card's appearance or preview the card in different states. Note that if null is provided, interactions will still happen internally. |
content | The content displayed on the card |
Code Examples
ElevatedCardSample
@Preview
@Composable
fun ElevatedCardSample() {
ElevatedCard(Modifier.size(width = 180.dp, height = 100.dp)) {
Box(Modifier.fillMaxSize()) { Text("Card content", Modifier.align(Alignment.Center)) }
}
}
ClickableElevatedCardSample
@Preview
@Composable
fun ClickableElevatedCardSample() {
ElevatedCard(
onClick = { /* Do something */ },
modifier = Modifier.size(width = 180.dp, height = 100.dp)
) {
Box(Modifier.fillMaxSize()) { Text("Clickable", Modifier.align(Alignment.Center)) }
}
}