TimePicker
Common
Component in Material 3 Compose
Time pickers help users select and set a specific time.
Shows a picker that allows the user to select time. Subscribe to updates through [TimePickerState]
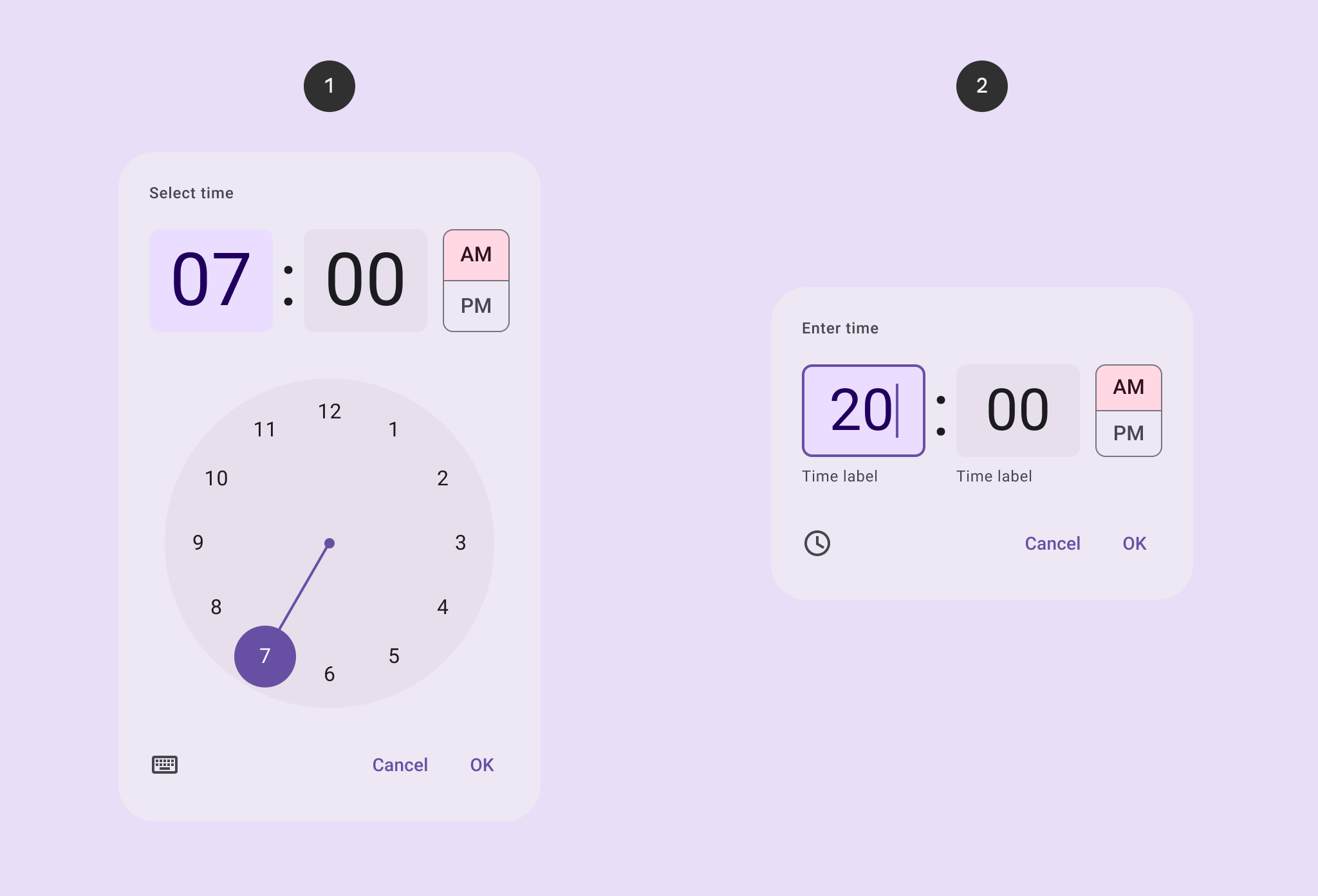
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha02")
}
Overloads
@Composable
@ExperimentalMaterial3Api
fun TimePicker(
state: TimePickerState,
modifier: Modifier = Modifier,
colors: TimePickerColors = TimePickerDefaults.colors(),
layoutType: TimePickerLayoutType = TimePickerDefaults.layoutType(),
)
Parameters
name | description |
---|---|
state | state for this time input, allows to subscribe to changes to [TimePickerState.hour] and [TimePickerState.minute], and set the initial time for this input. |
modifier | the [Modifier] to be applied to this time input |
colors | colors [TimePickerColors] that will be used to resolve the colors used for this time picker in different states. See [TimePickerDefaults.colors]. |
layoutType, | the different [TimePickerLayoutType] supported by this time picker, it will change the position and sizing of different components of the timepicker. |
Code Examples
TimePickerSample
@OptIn(ExperimentalMaterial3Api::class)
@Composable
@Preview
fun TimePickerSample() {
var showTimePicker by remember { mutableStateOf(false) }
val state = rememberTimePickerState()
val formatter = remember { SimpleDateFormat("hh:mm a", Locale.getDefault()) }
val snackState = remember { SnackbarHostState() }
val snackScope = rememberCoroutineScope()
Box(propagateMinConstraints = false) {
Button(modifier = Modifier.align(Alignment.Center), onClick = { showTimePicker = true }) {
Text("Set Time")
}
SnackbarHost(hostState = snackState)
}
if (showTimePicker) {
TimePickerDialog(
onCancel = { showTimePicker = false },
onConfirm = {
val cal = Calendar.getInstance()
cal.set(Calendar.HOUR_OF_DAY, state.hour)
cal.set(Calendar.MINUTE, state.minute)
cal.isLenient = false
snackScope.launch {
snackState.showSnackbar("Entered time: ${formatter.format(cal.time)}")
}
showTimePicker = false
},
) {
TimePicker(state = state)
}
}
}
TimePickerSwitchableSample
@OptIn(ExperimentalMaterial3Api::class)
@Composable
@Preview
fun TimePickerSwitchableSample() {
var showTimePicker by remember { mutableStateOf(false) }
val state = rememberTimePickerState()
val formatter = remember { SimpleDateFormat("hh:mm a", Locale.getDefault()) }
val snackState = remember { SnackbarHostState() }
val showingPicker = remember { mutableStateOf(true) }
val snackScope = rememberCoroutineScope()
val configuration = LocalConfiguration.current
Box(propagateMinConstraints = false) {
Button(modifier = Modifier.align(Alignment.Center), onClick = { showTimePicker = true }) {
Text("Set Time")
}
SnackbarHost(hostState = snackState)
}
if (showTimePicker) {
TimePickerDialog(
title =
if (showingPicker.value) {
"Select Time "
} else {
"Enter Time"
},
onCancel = { showTimePicker = false },
onConfirm = {
val cal = Calendar.getInstance()
cal.set(Calendar.HOUR_OF_DAY, state.hour)
cal.set(Calendar.MINUTE, state.minute)
cal.isLenient = false
snackScope.launch {
snackState.showSnackbar("Entered time: ${formatter.format(cal.time)}")
}
showTimePicker = false
},
toggle = {
if (configuration.screenHeightDp > 400) {
IconButton(onClick = { showingPicker.value = !showingPicker.value }) {
val icon =
if (showingPicker.value) {
Icons.Outlined.Keyboard
} else {
Icons.Outlined.Schedule
}
Icon(
icon,
contentDescription =
if (showingPicker.value) {
"Switch to Text Input"
} else {
"Switch to Touch Input"
}
)
}
}
}
) {
if (showingPicker.value && configuration.screenHeightDp > 400) {
TimePicker(state = state)
} else {
TimeInput(state = state)
}
}
}
}