DateRangePicker
Common
Component in Material 3 Compose
Material Design date range picker
Date range pickers let people select a range of dates and can be embedded into Dialogs.
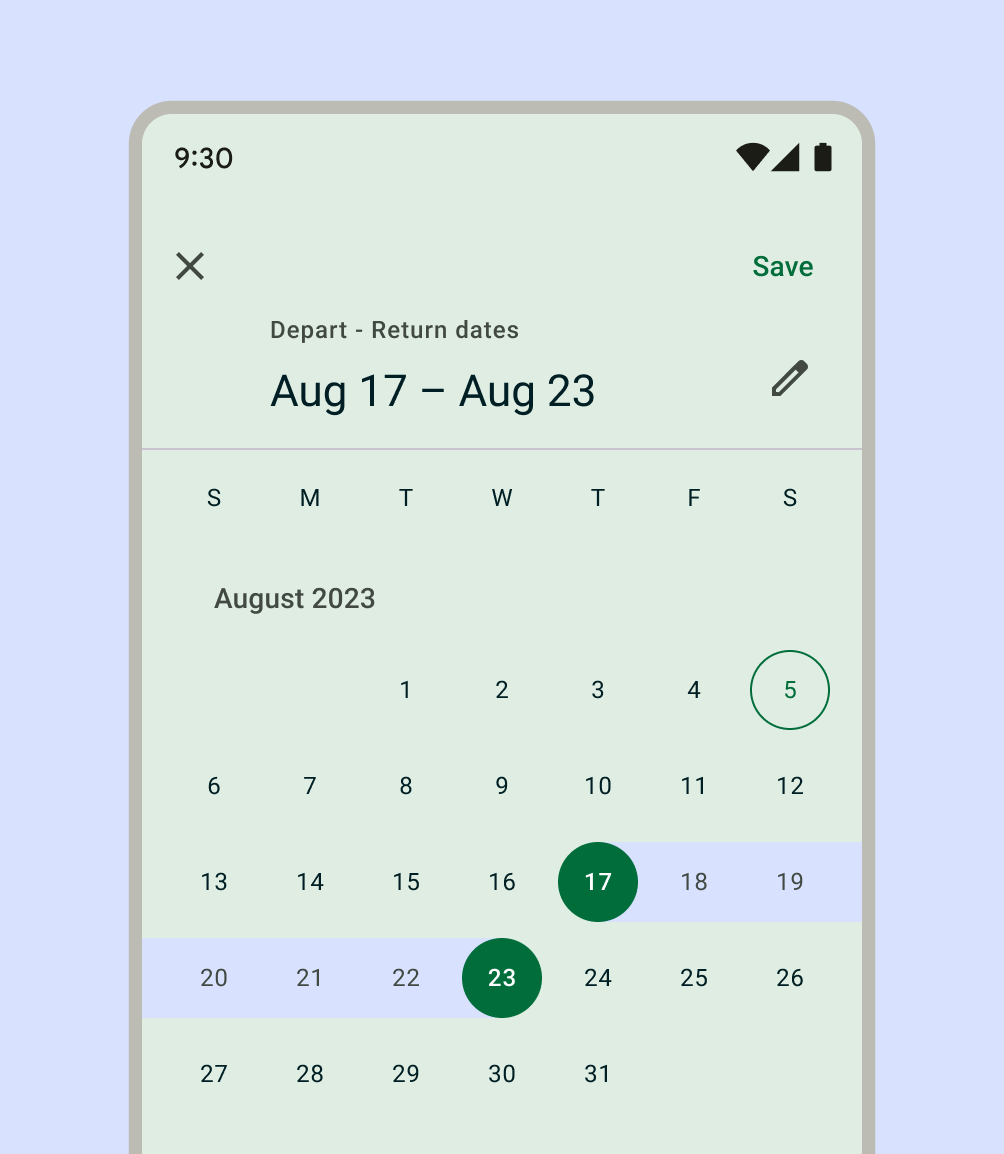
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha13")
}
Overloads
@ExperimentalMaterial3Api
@Composable
fun DateRangePicker(
state: DateRangePickerState,
modifier: Modifier = Modifier,
dateFormatter: DatePickerFormatter = remember { DatePickerDefaults.dateFormatter() },
colors: DatePickerColors = DatePickerDefaults.colors(),
title: (@Composable () -> Unit)? = {
DateRangePickerDefaults.DateRangePickerTitle(
displayMode = state.displayMode,
modifier = Modifier.padding(DateRangePickerTitlePadding),
contentColor = colors.titleContentColor
)
},
headline: (@Composable () -> Unit)? = {
DateRangePickerDefaults.DateRangePickerHeadline(
selectedStartDateMillis = state.selectedStartDateMillis,
selectedEndDateMillis = state.selectedEndDateMillis,
displayMode = state.displayMode,
dateFormatter,
modifier = Modifier.padding(DateRangePickerHeadlinePadding),
contentColor = colors.headlineContentColor
)
},
showModeToggle: Boolean = true,
focusRequester: FocusRequester? = remember { FocusRequester() }
)
Parameters
name | description |
---|---|
state | state of the date range picker. See [rememberDateRangePickerState]. |
modifier | the [Modifier] to be applied to this date range picker |
dateFormatter | a [DatePickerFormatter] that provides formatting skeletons for dates display |
colors | [DatePickerColors] that will be used to resolve the colors used for this date range picker in different states. See [DatePickerDefaults.colors]. |
title | the title to be displayed in the date range picker |
headline | the headline to be displayed in the date range picker |
showModeToggle | indicates if this DateRangePicker should show a mode toggle action that transforms it into a date range input |
focusRequester | a focus requester that will be used to focus the text field when the date picker is in an input mode. Pass null to not focus the text field if that's the desired behavior. |
@Deprecated(
message =
"Maintained for binary compatibility. Use the DateRangePicker with the focusRequester " +
"parameter.",
level = DeprecationLevel.HIDDEN
)
@ExperimentalMaterial3Api
@Composable
fun DateRangePicker(
state: DateRangePickerState,
modifier: Modifier = Modifier,
dateFormatter: DatePickerFormatter = remember { DatePickerDefaults.dateFormatter() },
colors: DatePickerColors = DatePickerDefaults.colors(),
title: (@Composable () -> Unit)? = {
DateRangePickerDefaults.DateRangePickerTitle(
displayMode = state.displayMode,
modifier = Modifier.padding(DateRangePickerTitlePadding),
contentColor = colors.titleContentColor
)
},
headline: (@Composable () -> Unit)? = {
DateRangePickerDefaults.DateRangePickerHeadline(
selectedStartDateMillis = state.selectedStartDateMillis,
selectedEndDateMillis = state.selectedEndDateMillis,
displayMode = state.displayMode,
dateFormatter,
modifier = Modifier.padding(DateRangePickerHeadlinePadding),
contentColor = colors.headlineContentColor
)
},
showModeToggle: Boolean = true,
requestFocus: Boolean = true
)
Parameters
name | description |
---|---|
state | state of the date range picker. See [rememberDateRangePickerState]. |
modifier | the [Modifier] to be applied to this date range picker |
dateFormatter | a [DatePickerFormatter] that provides formatting skeletons for dates display |
colors | [DatePickerColors] that will be used to resolve the colors used for this date range picker in different states. See [DatePickerDefaults.colors]. |
title | the title to be displayed in the date range picker |
headline | the headline to be displayed in the date range picker |
showModeToggle | indicates if this DateRangePicker should show a mode toggle action that transforms it into a date range input |
requestFocus | have a focus request be sent to the text field when the date picker is in an input mode |
Code Example
DateRangePickerSample
@OptIn(ExperimentalMaterial3Api::class)
@Preview
@Composable
fun DateRangePickerSample() {
// Decoupled snackbar host state from scaffold state for demo purposes.
val snackState = remember { SnackbarHostState() }
val snackScope = rememberCoroutineScope()
SnackbarHost(hostState = snackState, Modifier.zIndex(1f))
val state = rememberDateRangePickerState()
Column(modifier = Modifier.fillMaxSize(), verticalArrangement = Arrangement.Top) {
// Add a row with "Save" and dismiss actions.
Row(
modifier =
Modifier.fillMaxWidth()
.background(DatePickerDefaults.colors().containerColor)
.padding(start = 12.dp, end = 12.dp),
verticalAlignment = Alignment.CenterVertically,
horizontalArrangement = Arrangement.SpaceBetween
) {
IconButton(onClick = { /* dismiss the UI */ }) {
Icon(Icons.Filled.Close, contentDescription = "Localized description")
}
TextButton(
onClick = {
snackScope.launch {
val range = state.selectedStartDateMillis!!..state.selectedEndDateMillis!!
snackState.showSnackbar("Saved range (timestamps): $range")
}
},
enabled = state.selectedEndDateMillis != null
) {
Text(text = "Save")
}
}
DateRangePicker(state = state, modifier = Modifier.weight(1f))
}
}