OutlinedTextField
Common
Component in Material 3 Compose
Text fields allow users to enter text into a UI. They typically appear in forms and dialogs. Outlined text fields have less visual emphasis than filled text fields. When they appear in places like forms, where many text fields are placed together, their reduced emphasis helps simplify the layout.
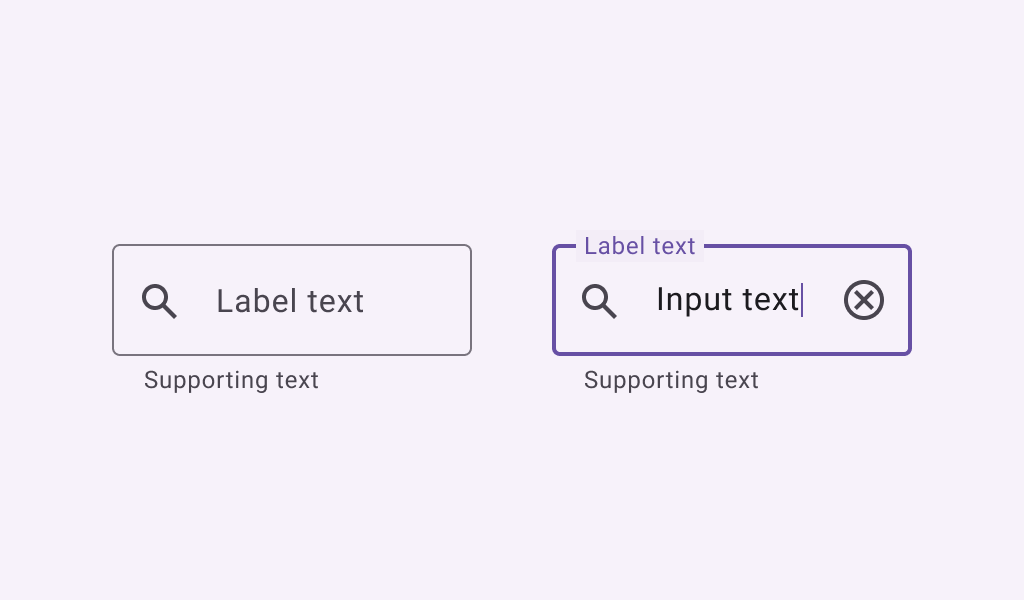
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha02")
}
Overloads
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextField(
state: TextFieldState,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
labelPosition: TextFieldLabelPosition = TextFieldLabelPosition.Default(),
label: @Composable (TextFieldLabelScope.() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
prefix: @Composable (() -> Unit)? = null,
suffix: @Composable (() -> Unit)? = null,
supportingText: @Composable (() -> Unit)? = null,
isError: Boolean = false,
inputTransformation: InputTransformation? = null,
outputTransformation: OutputTransformation? = null,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
onKeyboardAction: KeyboardActionHandler? = null,
lineLimits: TextFieldLineLimits = TextFieldLineLimits.Default,
onTextLayout: (Density.(getResult: () -> TextLayoutResult?) -> Unit)? = null,
scrollState: ScrollState = rememberScrollState(),
shape: Shape = OutlinedTextFieldDefaults.shape,
colors: TextFieldColors = OutlinedTextFieldDefaults.colors(),
contentPadding: PaddingValues = OutlinedTextFieldDefaults.contentPadding(),
interactionSource: MutableInteractionSource? = null,
)
Parameters
name | description |
---|---|
state | [TextFieldState] object that holds the internal editing state of the text field. |
modifier | the [Modifier] to be applied to this text field. |
enabled | controls the enabled state of this text field. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
readOnly | controls the editable state of the text field. When true , the text field cannot be modified. However, a user can focus it and copy text from it. Read-only text fields are usually used to display pre-filled forms that a user cannot edit. |
textStyle | the style to be applied to the input text. Defaults to [LocalTextStyle]. |
labelPosition | the position of the label. See [TextFieldLabelPosition]. |
label | the optional label to be displayed with this text field. The default text style uses [Typography.bodySmall] when minimized and [Typography.bodyLarge] when expanded. |
placeholder | the optional placeholder to be displayed when the input text is empty. The default text style uses [Typography.bodyLarge]. |
leadingIcon | the optional leading icon to be displayed at the beginning of the text field container. |
trailingIcon | the optional trailing icon to be displayed at the end of the text field container. |
prefix | the optional prefix to be displayed before the input text in the text field. |
suffix | the optional suffix to be displayed after the input text in the text field. |
supportingText | the optional supporting text to be displayed below the text field. |
isError | indicates if the text field's current value is in error. When true , the components of the text field will be displayed in an error color, and an error will be announced to accessibility services. |
inputTransformation | optional [InputTransformation] that will be used to transform changes to the [TextFieldState] made by the user. The transformation will be applied to changes made by hardware and software keyboard events, pasting or dropping text, accessibility services, and tests. The transformation will not be applied when changing the [state] programmatically, or when the transformation is changed. If the transformation is changed on an existing text field, it will be applied to the next user edit. The transformation will not immediately affect the current [state]. |
outputTransformation | optional [OutputTransformation] that transforms how the contents of the text field are presented. |
keyboardOptions | software keyboard options that contains configuration such as [KeyboardType] and [ImeAction]. |
onKeyboardAction | called when the user presses the action button in the input method editor (IME), or by pressing the enter key on a hardware keyboard. By default this parameter is null, and would execute the default behavior for a received IME Action e.g., [ImeAction.Done] would close the keyboard, [ImeAction.Next] would switch the focus to the next focusable item on the screen. |
lineLimits | whether the text field should be [SingleLine], scroll horizontally, and ignore newlines; or [MultiLine] and grow and scroll vertically. If [SingleLine] is passed, all newline characters ('\n') within the text will be replaced with regular whitespace (' '). |
onTextLayout | Callback that is executed when the text layout becomes queryable. The callback receives a function that returns a [TextLayoutResult] if the layout can be calculated, or null if it cannot. The function reads the layout result from a snapshot state object, and will invalidate its caller when the layout result changes. A [TextLayoutResult] object contains paragraph information, size of the text, baselines and other details. [Density] scope is the one that was used while creating the given text layout. |
scrollState | scroll state that manages either horizontal or vertical scroll of the text field. If [lineLimits] is [SingleLine], this text field is treated as single line with horizontal scroll behavior. Otherwise, the text field becomes vertically scrollable. |
shape | defines the shape of this text field's border. |
colors | [TextFieldColors] that will be used to resolve the colors used for this text field in different states. See [OutlinedTextFieldDefaults.colors]. |
contentPadding | the padding applied to the inner text field that separates it from the surrounding elements of the text field. Note that the padding values may not be respected if they are incompatible with the text field's size constraints or layout. See [OutlinedTextFieldDefaults.contentPadding]. |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this text field. You can use this to change the text field's appearance or preview the text field in different states. Note that if null is provided, interactions will still happen internally. |
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextField(
value: String,
onValueChange: (String) -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
label: @Composable (() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
prefix: @Composable (() -> Unit)? = null,
suffix: @Composable (() -> Unit)? = null,
supportingText: @Composable (() -> Unit)? = null,
isError: Boolean = false,
visualTransformation: VisualTransformation = VisualTransformation.None,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
keyboardActions: KeyboardActions = KeyboardActions.Default,
singleLine: Boolean = false,
maxLines: Int = if (singleLine) 1 else Int.MAX_VALUE,
minLines: Int = 1,
interactionSource: MutableInteractionSource? = null,
shape: Shape = OutlinedTextFieldDefaults.shape,
colors: TextFieldColors = OutlinedTextFieldDefaults.colors()
)
Parameters
name | description |
---|---|
value | the input text to be shown in the text field |
onValueChange | the callback that is triggered when the input service updates the text. An updated text comes as a parameter of the callback |
modifier | the [Modifier] to be applied to this text field |
enabled | controls the enabled state of this text field. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
readOnly | controls the editable state of the text field. When true , the text field cannot be modified. However, a user can focus it and copy text from it. Read-only text fields are usually used to display pre-filled forms that a user cannot edit. |
textStyle | the style to be applied to the input text. Defaults to [LocalTextStyle]. |
label | the optional label to be displayed with this text field. The default text style uses [Typography.bodySmall] when minimized and [Typography.bodyLarge] when expanded. |
placeholder | the optional placeholder to be displayed when the text field is in focus and the input text is empty. The default text style for internal [Text] is [Typography.bodyLarge] |
leadingIcon | the optional leading icon to be displayed at the beginning of the text field container |
trailingIcon | the optional trailing icon to be displayed at the end of the text field container |
prefix | the optional prefix to be displayed before the input text in the text field |
suffix | the optional suffix to be displayed after the input text in the text field |
supportingText | the optional supporting text to be displayed below the text field |
isError | indicates if the text field's current value is in error. If set to true, the label, bottom indicator and trailing icon by default will be displayed in error color |
visualTransformation | transforms the visual representation of the input [value] For example, you can use [PasswordVisualTransformation][androidx.compose.ui.text.input.PasswordVisualTransformation] to create a password text field. By default, no visual transformation is applied. |
keyboardOptions | software keyboard options that contains configuration such as [KeyboardType] and [ImeAction] |
keyboardActions | when the input service emits an IME action, the corresponding callback is called. Note that this IME action may be different from what you specified in [KeyboardOptions.imeAction] |
singleLine | when true , this text field becomes a single horizontally scrolling text field instead of wrapping onto multiple lines. The keyboard will be informed to not show the return key as the [ImeAction]. Note that [maxLines] parameter will be ignored as the maxLines attribute will be automatically set to 1. |
maxLines | the maximum height in terms of maximum number of visible lines. It is required that 1 {'<='} [minLines] {'<='} [maxLines]. This parameter is ignored when [singleLine] is true. |
minLines | the minimum height in terms of minimum number of visible lines. It is required that 1 {'<='} [minLines] {'<='} [maxLines]. This parameter is ignored when [singleLine] is true. |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this text field. You can use this to change the text field's appearance or preview the text field in different states. Note that if null is provided, interactions will still happen internally. |
shape | defines the shape of this text field's border |
colors | [TextFieldColors] that will be used to resolve the colors used for this text field in different states. See [OutlinedTextFieldDefaults.colors]. |
@OptIn(ExperimentalMaterial3Api::class)
@Composable
fun OutlinedTextField(
value: TextFieldValue,
onValueChange: (TextFieldValue) -> Unit,
modifier: Modifier = Modifier,
enabled: Boolean = true,
readOnly: Boolean = false,
textStyle: TextStyle = LocalTextStyle.current,
label: @Composable (() -> Unit)? = null,
placeholder: @Composable (() -> Unit)? = null,
leadingIcon: @Composable (() -> Unit)? = null,
trailingIcon: @Composable (() -> Unit)? = null,
prefix: @Composable (() -> Unit)? = null,
suffix: @Composable (() -> Unit)? = null,
supportingText: @Composable (() -> Unit)? = null,
isError: Boolean = false,
visualTransformation: VisualTransformation = VisualTransformation.None,
keyboardOptions: KeyboardOptions = KeyboardOptions.Default,
keyboardActions: KeyboardActions = KeyboardActions.Default,
singleLine: Boolean = false,
maxLines: Int = if (singleLine) 1 else Int.MAX_VALUE,
minLines: Int = 1,
interactionSource: MutableInteractionSource? = null,
shape: Shape = OutlinedTextFieldDefaults.shape,
colors: TextFieldColors = OutlinedTextFieldDefaults.colors()
)
Parameters
name | description |
---|---|
value | the input [TextFieldValue] to be shown in the text field |
onValueChange | the callback that is triggered when the input service updates values in [TextFieldValue]. An updated [TextFieldValue] comes as a parameter of the callback |
modifier | the [Modifier] to be applied to this text field |
enabled | controls the enabled state of this text field. When false , this component will not respond to user input, and it will appear visually disabled and disabled to accessibility services. |
readOnly | controls the editable state of the text field. When true , the text field cannot be modified. However, a user can focus it and copy text from it. Read-only text fields are usually used to display pre-filled forms that a user cannot edit. |
textStyle | the style to be applied to the input text. Defaults to [LocalTextStyle]. |
label | the optional label to be displayed with this text field. The default text style uses [Typography.bodySmall] when minimized and [Typography.bodyLarge] when expanded. |
placeholder | the optional placeholder to be displayed when the text field is in focus and the input text is empty. The default text style for internal [Text] is [Typography.bodyLarge] |
leadingIcon | the optional leading icon to be displayed at the beginning of the text field container |
trailingIcon | the optional trailing icon to be displayed at the end of the text field container |
prefix | the optional prefix to be displayed before the input text in the text field |
suffix | the optional suffix to be displayed after the input text in the text field |
supportingText | the optional supporting text to be displayed below the text field |
isError | indicates if the text field's current value is in error state. If set to true, the label, bottom indicator and trailing icon by default will be displayed in error color |
visualTransformation | transforms the visual representation of the input [value] For example, you can use [PasswordVisualTransformation][androidx.compose.ui.text.input.PasswordVisualTransformation] to create a password text field. By default, no visual transformation is applied. |
keyboardOptions | software keyboard options that contains configuration such as [KeyboardType] and [ImeAction] |
keyboardActions | when the input service emits an IME action, the corresponding callback is called. Note that this IME action may be different from what you specified in [KeyboardOptions.imeAction] |
singleLine | when true , this text field becomes a single horizontally scrolling text field instead of wrapping onto multiple lines. The keyboard will be informed to not show the return key as the [ImeAction]. Note that [maxLines] parameter will be ignored as the maxLines attribute will be automatically set to 1. |
maxLines | the maximum height in terms of maximum number of visible lines. It is required that 1 {'<='} [minLines] {'<='} [maxLines]. This parameter is ignored when [singleLine] is true. |
minLines | the minimum height in terms of minimum number of visible lines. It is required that 1 {'<='} [minLines] {'<='} [maxLines]. This parameter is ignored when [singleLine] is true. |
interactionSource | an optional hoisted [MutableInteractionSource] for observing and emitting [Interaction]s for this text field. You can use this to change the text field's appearance or preview the text field in different states. Note that if null is provided, interactions will still happen internally. |
shape | defines the shape of this text field's border |
colors | [TextFieldColors] that will be used to resolve the colors used for this text field in different states. See [OutlinedTextFieldDefaults.colors]. |
Code Examples
SimpleOutlinedTextFieldSample
@Preview
@Composable
fun SimpleOutlinedTextFieldSample() {
OutlinedTextField(
state = rememberTextFieldState(),
lineLimits = TextFieldLineLimits.SingleLine,
label = { Text("Label") },
)
}
OutlinedTextFieldWithInitialValueAndSelection
@Preview
@Composable
fun OutlinedTextFieldWithInitialValueAndSelection() {
val state = rememberTextFieldState("Initial text", TextRange(0, 12))
OutlinedTextField(
state = state,
lineLimits = TextFieldLineLimits.SingleLine,
label = { Text("Label") },
)
}