LoadingIndicator
Common
Component in Material 3 Compose
A Material Design loading indicator.
This version of the loading indicator morphs between its [polygons] shapes by the value of its [progress].
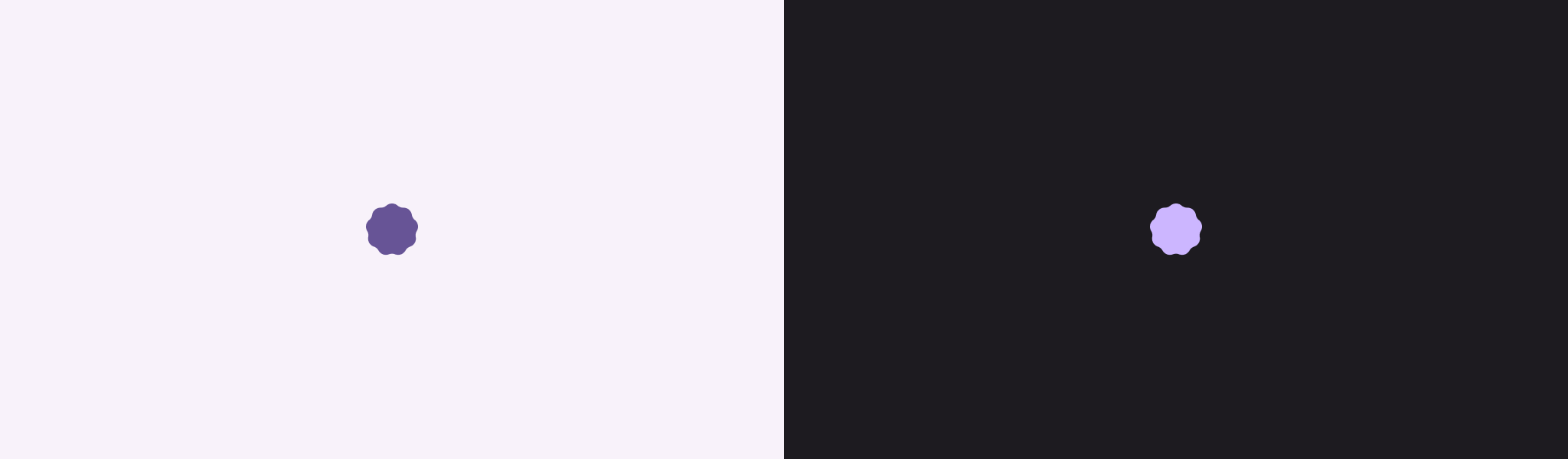
Last updated:
Installation
dependencies {
implementation("androidx.compose.material3:material3:1.4.0-alpha15")
}
Overloads
@ExperimentalMaterial3ExpressiveApi
@Composable
fun LoadingIndicator(
progress: () -> Float,
modifier: Modifier = Modifier,
color: Color = LoadingIndicatorDefaults.indicatorColor,
polygons: List<RoundedPolygon> = LoadingIndicatorDefaults.DeterminateIndicatorPolygons
)
Parameters
name | description |
---|---|
progress | the progress of this loading indicator, where 0.0 represents no progress and 1.0 represents full progress. Values outside of this range are coerced into the range. The indicator will morph its shapes between the provided [polygons] according to the value of the progress. |
modifier | the [Modifier] to be applied to this loading indicator |
color | the loading indicator's color |
polygons | a list of [RoundedPolygon]s for the sequence of shapes this loading indicator will morph between as it progresses from 0.0 to 1.0. The loading indicator expects at least two items in that list. |
@ExperimentalMaterial3ExpressiveApi
@Composable
fun LoadingIndicator(
modifier: Modifier = Modifier,
color: Color = LoadingIndicatorDefaults.indicatorColor,
polygons: List<RoundedPolygon> = LoadingIndicatorDefaults.IndeterminateIndicatorPolygons,
)
Parameters
name | description |
---|---|
modifier | the [Modifier] to be applied to this loading indicator |
color | the loading indicator's color |
polygons | a list of [RoundedPolygon]s for the sequence of shapes this loading indicator will morph between. The loading indicator expects at least two items in that list. |
Code Examples
DeterminateLoadingIndicatorSample
@OptIn(ExperimentalMaterial3ExpressiveApi::class)
@Preview
@Composable
fun DeterminateLoadingIndicatorSample() {
var progress by remember { mutableFloatStateOf(0f) }
val animatedProgress by
animateFloatAsState(
targetValue = progress,
animationSpec =
spring(
dampingRatio = Spring.DampingRatioNoBouncy,
stiffness = Spring.StiffnessVeryLow,
visibilityThreshold = 1 / 1000f
)
)
Column(horizontalAlignment = Alignment.CenterHorizontally) {
LoadingIndicator(progress = { animatedProgress })
Spacer(Modifier.requiredHeight(30.dp))
Text("Set loading progress:")
Slider(
modifier = Modifier.width(300.dp),
value = progress,
valueRange = 0f..1f,
onValueChange = { progress = it },
)
}
}
LoadingIndicatorSample
@OptIn(ExperimentalMaterial3ExpressiveApi::class)
@Preview
@Composable
fun LoadingIndicatorSample() {
Column(horizontalAlignment = Alignment.CenterHorizontally) { LoadingIndicator() }
}